ZIO HTTP
A next-generation Scala framework for building scalable, correct, and efficient HTTP clients and servers
Imperative API
Server-side Example
import zio._
import zio.http._
object GreetingServer extends ZIOAppDefault {
val routes =
Routes(
Method.GET / "greet" -> handler { (req: Request) =>
val name = req.queryOrElse("name", "World")
Response.text(s"Hello $name!")
}
)
def run = Server.serve(routes).provide(Server.default)
}
Client-side Example
import zio._
import zio.http._
import zio.http.codec.TextBinaryCodec.fromSchema
object ClientServerExample extends ZIOAppDefault {
val clientApp: ZIO[Client, Throwable, Unit] =
for {
url <- ZIO.fromEither(URL.decode("http://localhost:8080/greet"))
res <- ZClient.batched(
Request
.get(url)
.setQueryParams(
Map("name" -> Chunk("ZIO HTTP"))
)
)
body <- res.bodyAs[String]
_ <- ZIO.debug("Received response: " + body)
} yield ()
val run = clientApp.provide(Client.default)
}
Declarative API
// Endpoint Definition
val endpoint =
Endpoint(GET / "greet" ?? Doc.p("Route for querying books"))
.query(
HttpCodec.query[String]("name") ?? Doc.p("Name of the person to greet"),
)
.out[String]
// Endpoint Implementation
val greetRoute: Route[Any, Nothing] =
endpoint.implementHandler(handler((name: String) => s"Hello, $name!"))
Key Features
Build high-performance, scalable web applications with ZIO HTTP
High Performance & Non-blocking
ZIO HTTP is powered by Netty and ZIO's asynchronous runtime, so all I/O is event-driven and non-blocking. This yields extremely high throughput and low latency with minimal resource use.
Unified ZIO Experience
Built on ZIO's effect system, ZIO HTTP provides lightweight fibers, structured error handling, and resource safety for your web applications, with seamless access to ZIO ecosystem libraries, such as ZIO Schema, ZIO Logging, ZIO Config, ZIO Streams, and ZIO Test libraries.
Cloud-Native Support
Designed for cloud-scale deployments, ZIO HTTP supports massive concurrency and parallelism inherently. It efficiently manages thousands of fibers (lightweight threads) and connections, so your services can scale horizontally under load.
Type-driven Endpoints
ZIO HTTP supports both imperative routing and declarative, schema-driven endpoints. You describe request and response schemas in types and the framework type-checks your handler logic against them at compile time.
Ecosystem
A complete toolkit for building scalable, resilient applications
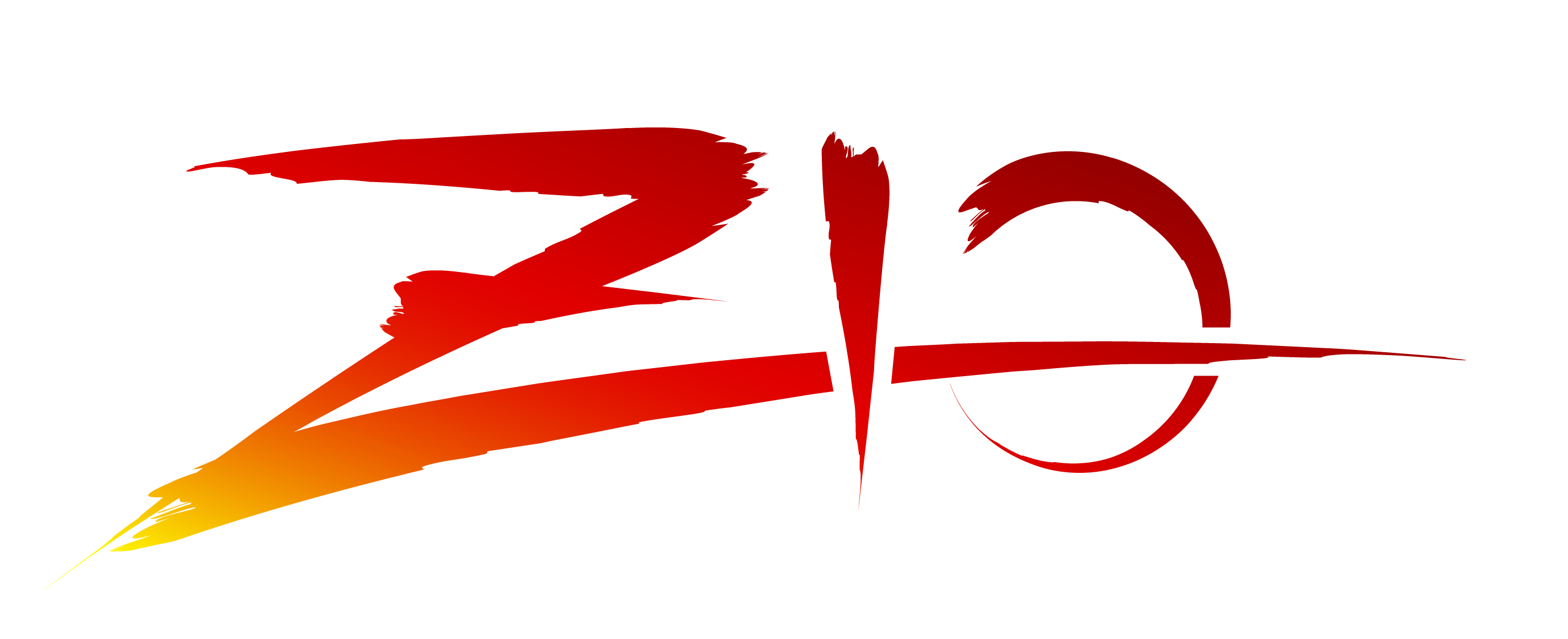
ZIO
A type-safe, composable library for async and concurrent programming in Scala
- Build scalable applications with minimal overhead.
- Catch bugs at compile time with powerful type safety.
- Write concurrent code without deadlocks or race conditions.
- Seamless code for both sync and async operations.
- Never leak resources, even during failures.
- Build resilient systems that handle errors gracefully.
- Compose complex solutions from simple building blocks.
- Built-in dependency injection.
- Structured concurrency with simplicity and safety.
- Fine-grained interruption for cancelable operations.
- Integrated metrics and observability.
- Seamless interop with Java and Scala libraries.
ZIO Schema
A library for describing data types and their encodings in a composable way
- Automatic derivation of schemas
- Support for many formats (JSON, Protobuf, etc.)
- Schema transformations and migrations
- Integration with ZIO HTTP
ZIO Config
A type-safe, composable library for configuration management in Scala
- Type-safe configuration descriptions
- Multiple source support (files, env vars, etc.)
- Documentation generation
- Validation and error reporting
ZIO Logging
A composable logging library for ZIO applications
- Structured logging for ZIO applications
- Multiple backend support
- Context-aware logging
- Integration with MDC and log correlation
ZIO Stream
A powerful streaming library for processing infinite data with finite resources
- Fully asynchronous and non-blocking streaming
- Resource-safe processing of large data
- Rich set of combinators for data transformation
- Backpressure handling and flow control
ZIO Test
A testing framework built on ZIO for writing comprehensive, concurrent tests
- Property-based testing
- First-class support for asynchronous testing
- Compositional test aspects for reusable configurations
- Run test effects in parallel for faster test suites
- Integration with JUnit and other test frameworks
ZIO STM
Software Transactional Memory for composable concurrent state management
- Atomic, isolated transactions
- Composable concurrent operations
- No deadlocks or race conditions
- Automatic retry of interrupted transactions
Learn ZIO HTTP with Zionomicon
The comprehensive guide to building scalable applications with ZIO
Zionomicon is the definitive guide to ZIO, written by the creators of the library. It covers all aspects of the ZIO ecosystem, including a dedicated chapter on ZIO HTTP that teaches you how to build high-performance, type-safe web applications and APIs.
In the ZIO HTTP chapter, you'll learn:
- Building scalable HTTP servers with minimal boilerplate
- How ZIO HTTP is modeled as "HTTP as a Function"
- Type-safe request handling
- Working with middleware and interceptors
- How to design your API declaratively
Who are Using ZIO HTTP?
Organizations and projects building with ZIO HTTP in production
Are you using ZIO HTTP? Let us know and join the list!